Quickstart
Overview
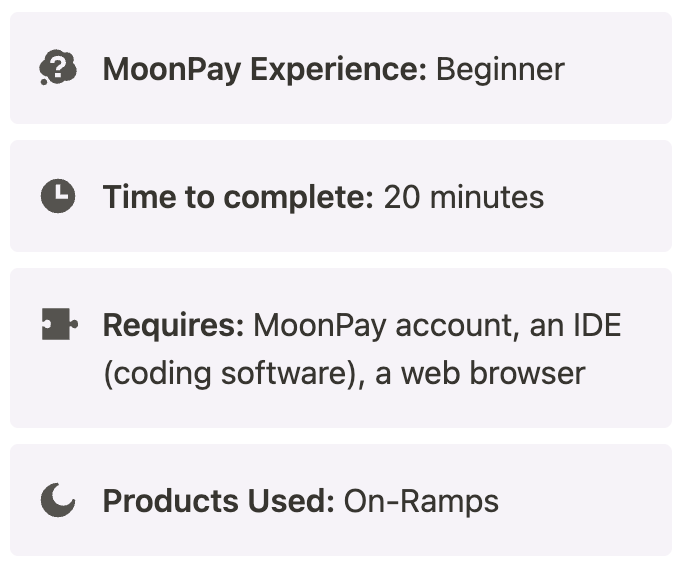
In this Quickstart, you will facilitate cryptocurrency purchases via the MoonPay On-Ramp directly from your browser.
Whether you are a complete beginner to coding or a seasoned expert, this guide will take you through all of the necessary steps to have you converting fiat to cryptocurrency in no time.
What you will accomplish
In this tutorial, you will:
- Configure On-Ramps widget in a local environment
- Create a new folder for code
- Initialize the widget in a file using HTML and JavaScript
- Edit the widget parameters
- Display the fully functional widget locally in your browser
Prerequisites
Before beginning, you will need:
- A MoonPay account: If you do not have one, set one up on this page.
- An IDE (coding software): Some popular ones are Visual Studio Code and IntelliJ WebStorm. We will be using Visual Studio Code in this tutorial.
- A web browser: Any web browser will work. We will be using Google Chrome in this tutorial.
Implementation
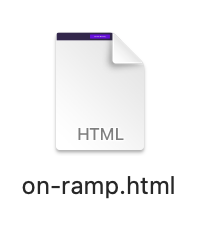
Step 1: Set up a file for your integration
In your IDE, create a new file for your On-Ramp code. Ours will be called on-ramp.html.
Step 2: Retrieve API keys from the MoonPay Dashboard
- Navigate to your MoonPay Dashboard and log in.
- Once logged in, navigate to the Developers tab on the sidebar → API Keys.
- Here, you will be able to copy your API keys. In this tutorial, we will be using the publishable test key.
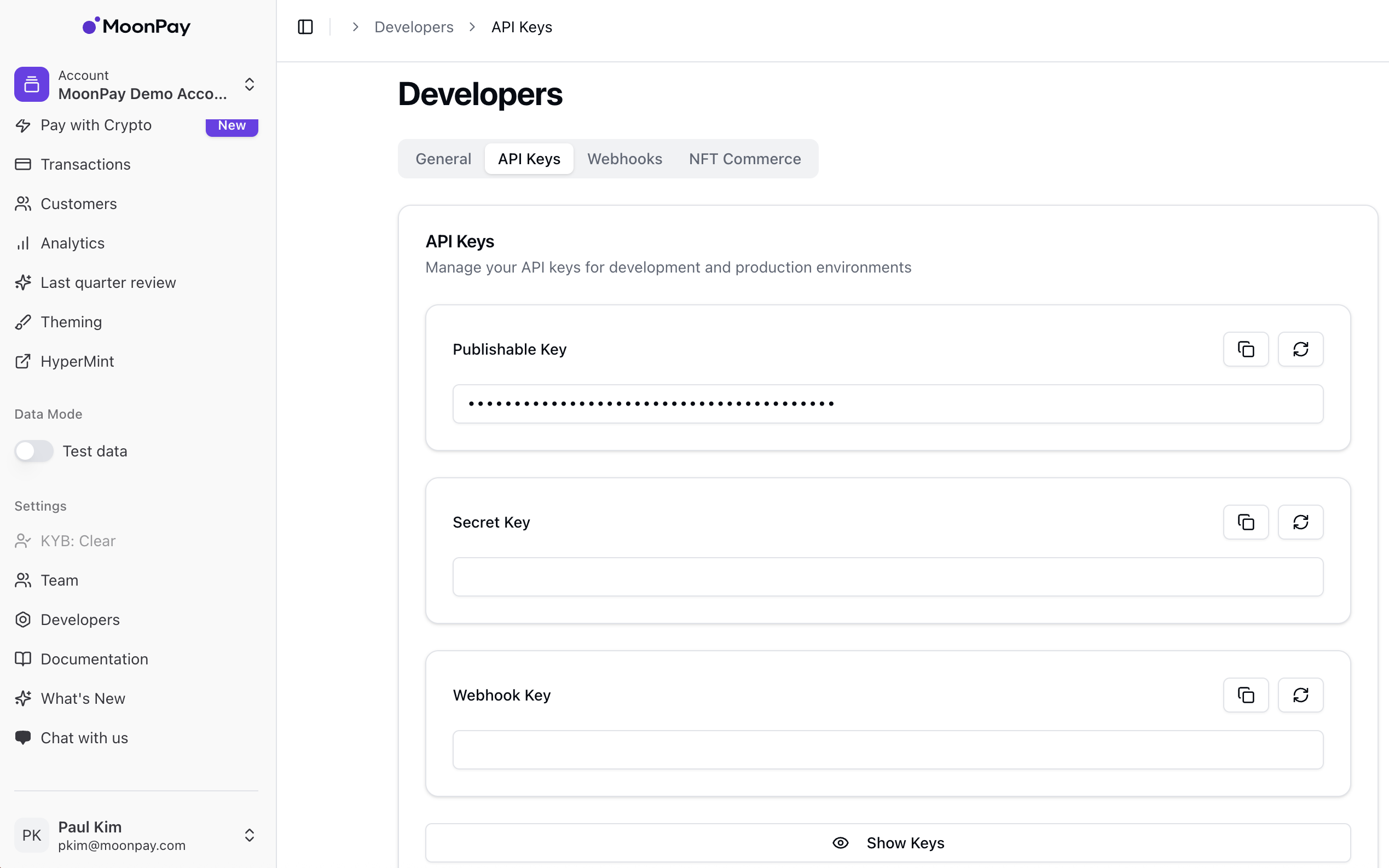
Step 3: Insert HTML and code into on-ramp.html
-
The code below will embed our Software Development Kit (SDK) directly into your HTML by way of
<script>
tags.- You can think of this as a way of injecting code directly into HTML without having to create a separate linked file
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>MoonPay Integration</title> <!-- Adds the MoonPay SDK as a script to your HTML file --> <script defer src="https://static.moonpay.com/web-sdk/v1/moonpay-web-sdk.min.js"></script> <!-- Initialize the MoonPay SDK immediately upon page load --> <script> document.addEventListener("DOMContentLoaded", () => { const moonpaySdk = window.MoonPayWebSdk.init({ flow: "buy", environment: "sandbox", variant: "overlay", params: { apiKey: "pk_test_123", // Replace with your MoonPay publishable API key baseCurrencyCode: "usd", baseCurrencyAmount: "30", defaultCurrencyCode: "eth" }}); // Open widget moonpaySdk.show(); }); </script> </head> <body> <div class="container"> </div> </body> </html>
-
This script will detect when the HTML page loads and immediately run the JavaScript within the
<script>
tags when the load has finished. Remember to replacepk_test
with your publishable API key from Step 1. -
You can customize widget functionality (currencies, amounts, etc.) by adding and removing parameters in the JavaScript, whilst still preserving proper formatting.
- All parameters can be found on this page of our documentation.
-
For example, if we would like to display the widget with a default of 100 GBP worth of BTC, and also specify the
walletAddress
in the parameters so that the user is not prompted for theirs, we could modify the JavaScript like such:
<script>
document.addEventListener("DOMContentLoaded", () => {
const moonpaySdk = window.MoonPayWebSdk.init({
flow: "buy",
environment: "sandbox",
variant: "overlay",
params: {
apiKey: "pk_test", // Replace with your MoonPay publishable API key
baseCurrencyCode: "gbp",
baseCurrencyAmount: "100",
defaultCurrencyCode: "btc",
walletAddress: "enter your wallet here"
}});
// Open widget
moonpaySdk.show();
});
</script>
Step 4: Do a test transaction
- The first step of processing a test transaction using the MoonPay On-Ramp is to make sure that
environment
is set to"sandbox"
, as shown above in the example code. This puts your widget in test mode. - Next, open up the widget, enter your email address, and log in.
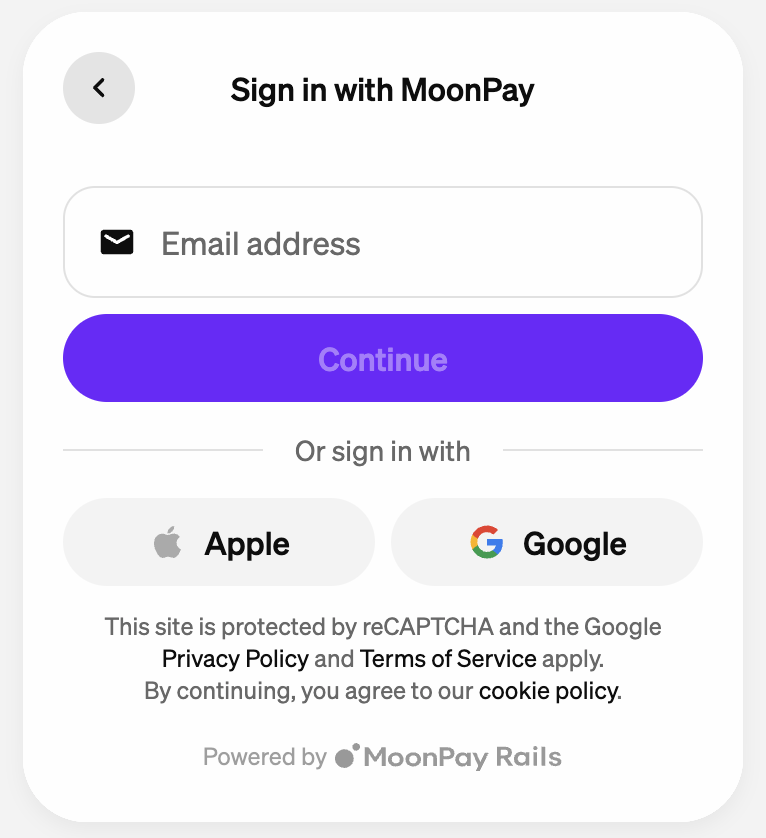
- Next, you will be asked to enter a wallet address if one hasn’t been passed through the parameters already. If you already have existing wallet addresses with MoonPay, they will show up here. Any wallet address will work for a test transaction.
- Next, go and grab the Test Credit Card from this page of our documentation.
Remember!
Never use a test credit card on anything other than a test widget that has environment: "sandbox", otherwise your MoonPay account may be blocked.
- If successful, you should see a screen that looks like the below.
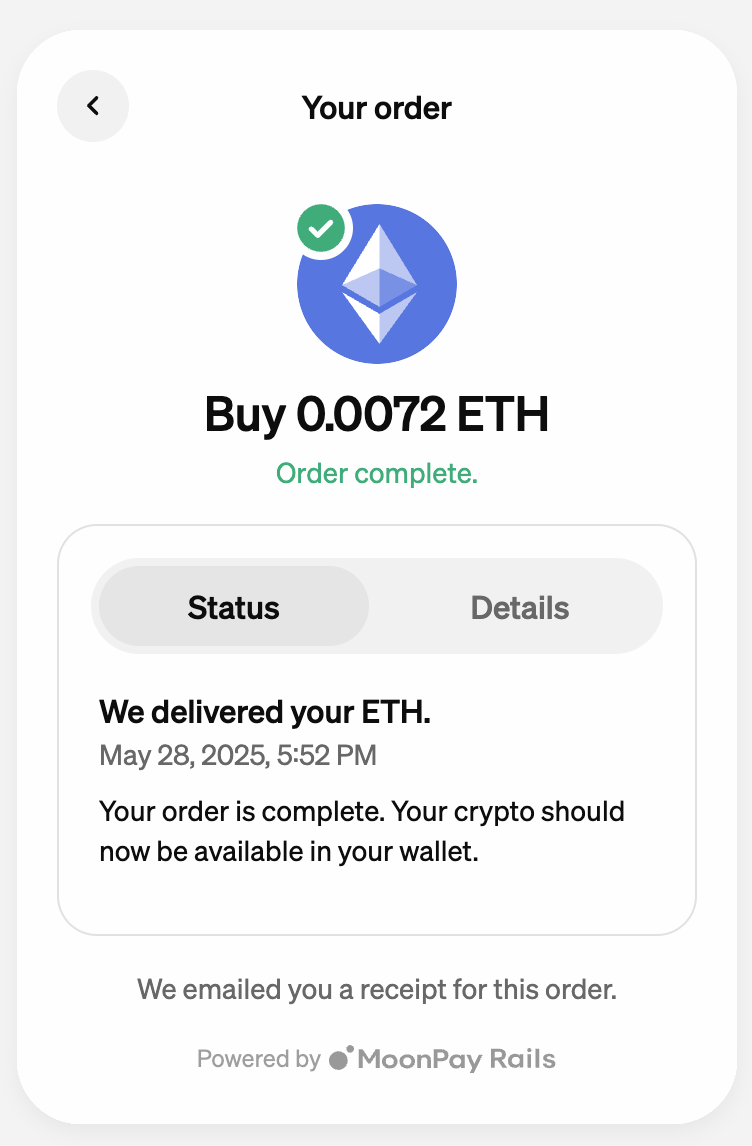
Completion
Congratulations, you have just integrated the MoonPay On-Ramp! 🎉
There are so many ways to integrate this widget to enhance user experience when buying cryptocurrency. If you want to know about alternative ways to integrate with MoonPay, check our Integrations (SDKs) page.
Any questions? Feel free to visit our Help Center to dig into our other resources or drop us a line!
Updated about 2 months ago