<PassGenerator />
Generated Passes from NFT Collections
If you specify contracts, the user will be presented with a list of all NFTs within the specified contract addresses. After selecting an NFT, the user will need to sign a message to validate ownership, which then allows the creation of a pass representing ownership of that NFT.
Usage
import { PassGenerator } from '@ethpass/sdk';
import { ethers } from 'ethers';
const provider = ethers.getDefaultProvider()
const NFTPassGenerator = () => {
return (
<PassGenerator
provider={provider}
contracts={[
{
address: '0x8765e43765fA715Af70A1e4483814660B48b497C',
},
]}
/>
);
};
export default NFTPassGenerator;
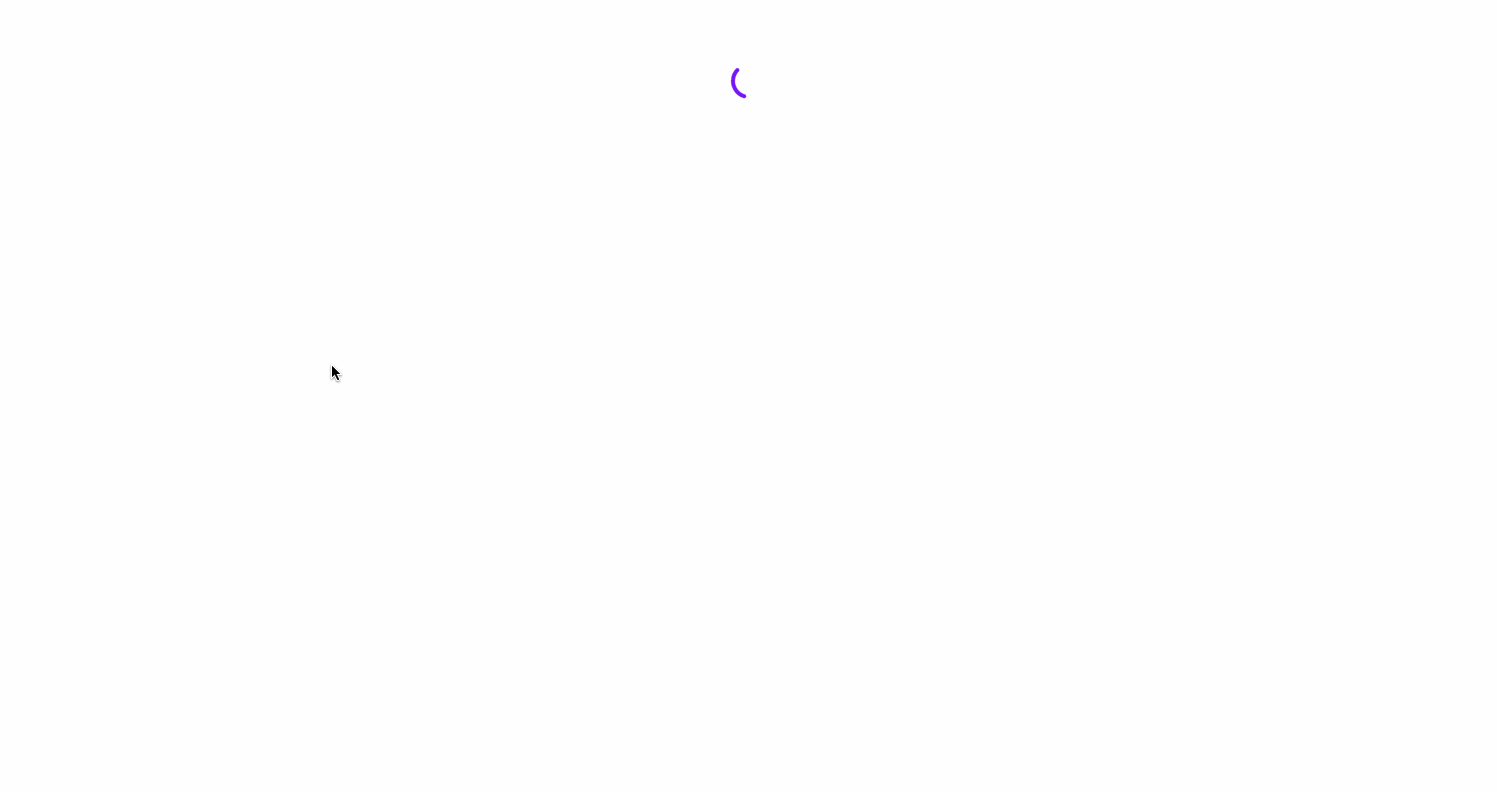
Generated Passes from Policies
By specifying an array of policyIds, the user will see a button to generate a pass, but only if their connected wallet meets the criteria set within the policy.
Usage
import { PassGenerator } from '@ethpass/sdk';
import { ethers } from 'ethers';
const provider = ethers.getDefaultProvider()
const PolicyPassGenerator = () => {
return (
<PassGenerator
provider={provider}
policyIds={['07d79222-4dc8-4a1d-bb1e-0ce5608f2125']}
showRequirements
/>
);
};
export default PolicyPassGenerator;
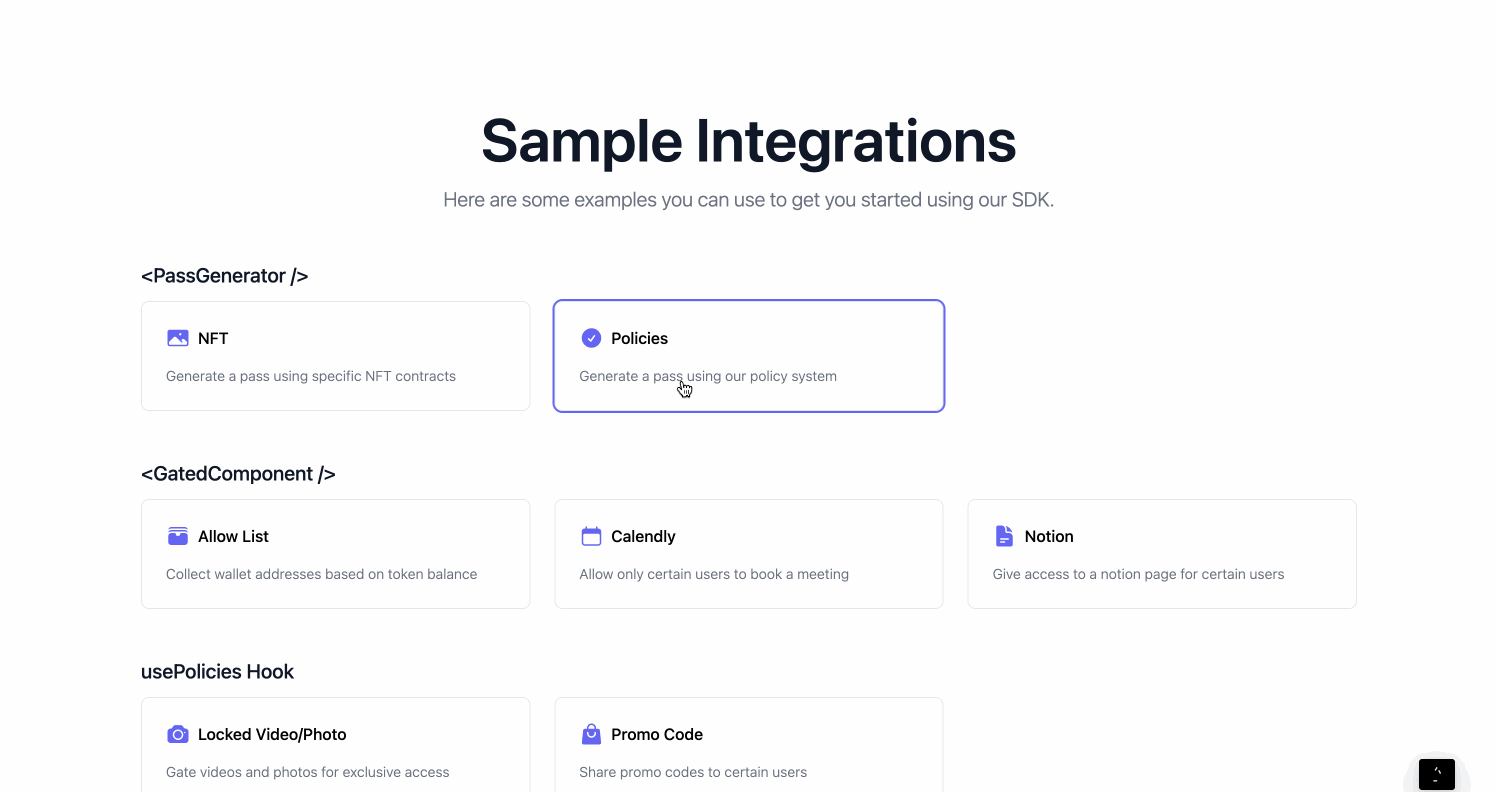
Props
⚠️ Note:
contracts
andpolicyIds
cannot be used simultaneously
Name | Type | Description |
---|---|---|
provider | Web3Provider | The Web3Provider instance. |
contracts | Array<{ address: string; slug?: never } | { address?: never; slug: string }> | undefined | An array of objects, each with an address or a slug property, but not both. slug property should be used for OpenSea contracts |
policyIds | Array<string> | An array of strings representing the identifiers of policies to be applied. |
layout | PassLayout | Optional. Specifies the layout of the pass. Should be an instance of PassLayout . |
signatureMessage | string | Optional. A custom message to be signed by the wallet during the generation process. |
templateId | string | Optional. The identifier for a specific template to be used in pass generation. |
showRequirements | boolean | Optional. A boolean flag to indicate whether requirements should be displayed. Defaults to false . |
checkDuplicateWallet | boolean | Optional. A boolean indicating whether to check for duplicate wallet addresses. Defaults to false . |
checkDuplicateToken | boolean | Optional. A boolean indicating whether to check for duplicate tokens. Defaults to false . |
Updated 11 months ago