Configure user authentication methods
This document provides an overview of different user authentication methods available in the MoonPay Authenticate SDK.
SDK configuration
const sdk = new MoonPayAuthSDK('your_publishable_key', {
devApiUrl: 'https://api.moonpay.com/graphql',
devAuthDomain: 'https://auth.moonpay.com/graphql',
// Login options:
components: [LoginComponents.EmailOtp, LoginComponents.SocialLogin],
oauthOptions: {
providers: [OAuthProviders.Google, OAuthProviders.Apple],
mode: 'horizontal', // Display mode can be 'horizontal' or 'vertical'.
appleAllowHideMyEmail: true, // Allows the "Hide My Email" feature for Apple OAuth.
},
linkVerifiedWallet: true, // Support linking crypto wallets.
// Embed instead of iFrame overlay.
embeddableOptions: {
elementId: 'custom-div',
width: 500,
height: 300,
},
})
Authentication methods
Email OTP
Email OTP (One-Time Password) is a method where users enter their email, receive a one-time password in their email inbox, and use that password to authenticate on a partner website.
Usage
First configure the SDK:
const sdk = new MoonPayAuthSDK('your_publishable_key', {
components: [LoginComponents.EmailLoginLink],
})
Then call the show
method:
sdk.login.show();
You can also prefill the user's email:
sdk.login.show({
email: '[email protected]'
});
Try out the demo here: Demo
Screenshots
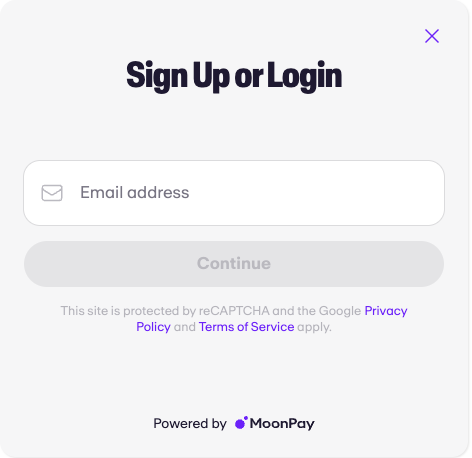
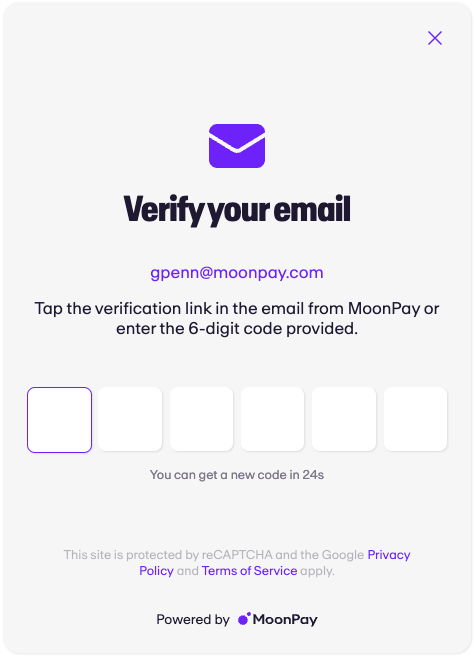
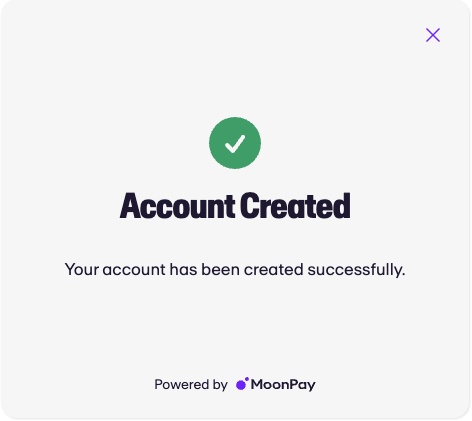
Login link
Login Link is a passwordless authentication method. Users enter their email, receive a link in their email, and clicking on the link automatically authenticates them.
Configuration
First configure the SDK:
const sdk = new MoonPayAuthSDK('your_publishable_key', {
components: [LoginComponents.EmailLoginLink],
})
Then call the show
method:
sdk.login.show();
You can also prefill the user's email:
sdk.login.show({
email: '[email protected]'
});
Try out the demo here: Demo
Screenshots
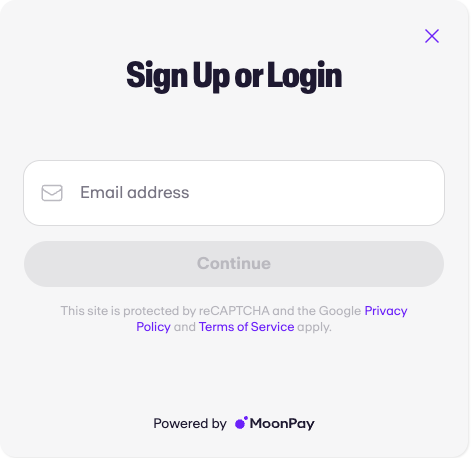
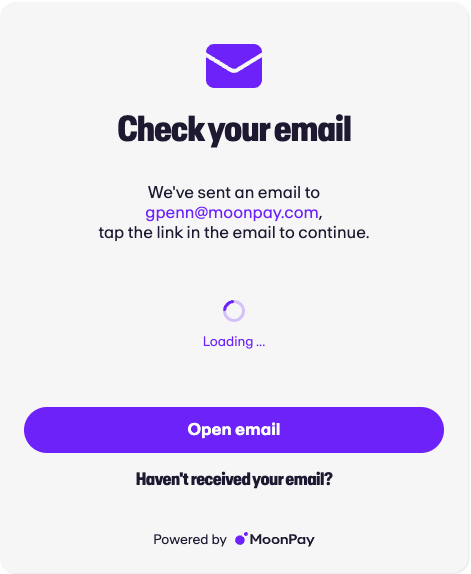
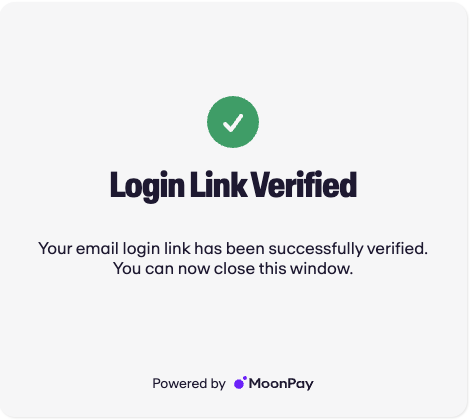
Apple and Google OAuth
MoonPay Authenticate SDK supports Apple and Google OAuth for seamless and secure user authentication. Follow the steps below to integrate these authentication providers into your application.
Configuration
Import the OAuthProviders
and OAuthOptions
code from the Auth SDK package:
import { OAuthProviders, OAuthOptions } from "@moonpay/auth-sdk";
Update your SDK configuration to enable SocialLogin
, and include Apple and/or Google as supported OAuth providers. Additionally, you can specify the display mode and Apple's "Hide My Email" option:
const sdk = new MoonPayAuthSDK('your_publishable_key', {
components: [LoginComponents.SocialLogin],
oauthOptions: {
providers: [OAuthProviders.Google, OAuthProviders.Apple],
mode: 'horizontal', // Display mode can be 'horizontal' or 'vertical'.
appleAllowHideMyEmail: true, // Allows the "Hide My Email" feature for Apple OAuth.
},
})
To show the modal with the OAuth methods, continue to use the same code, such as sdk.login.show()
Try out the demo here: Demo
Screenshots
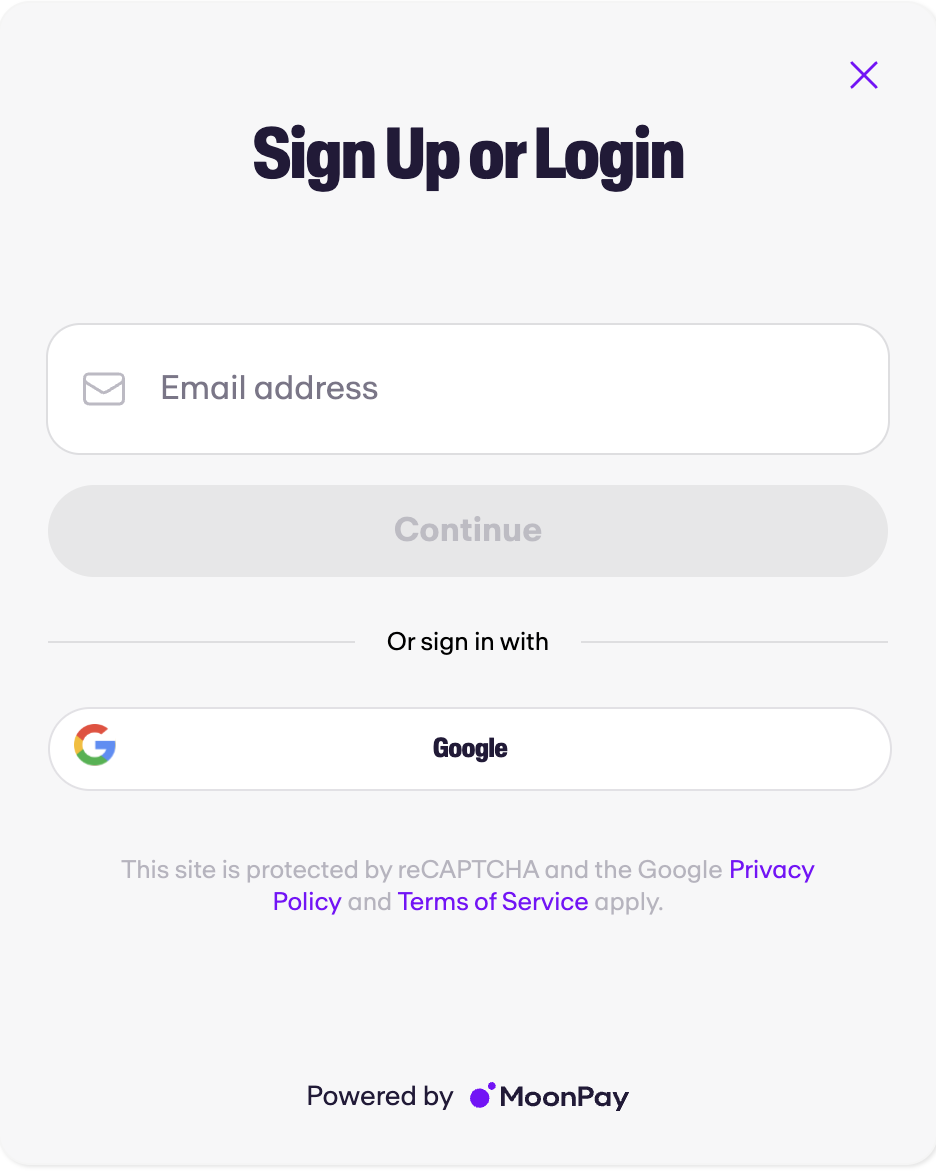
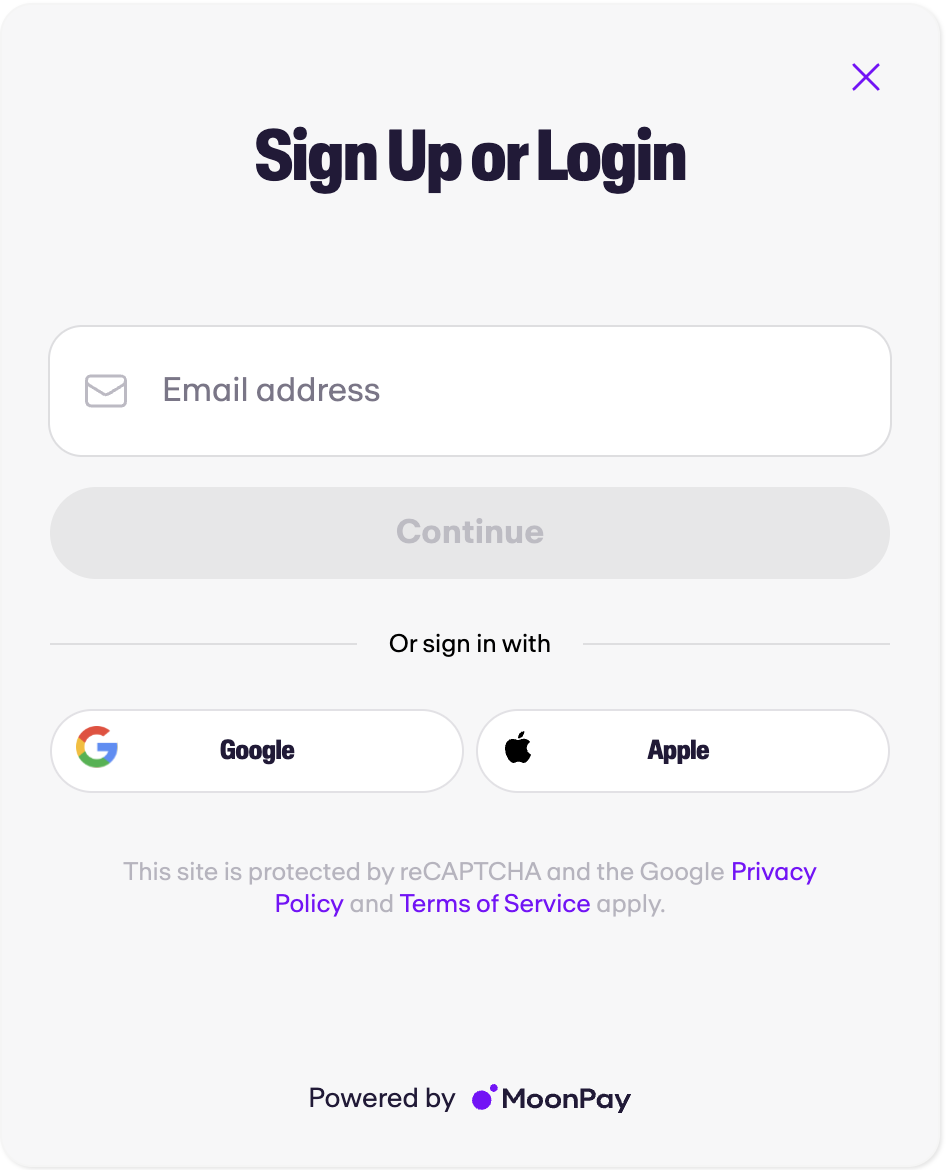
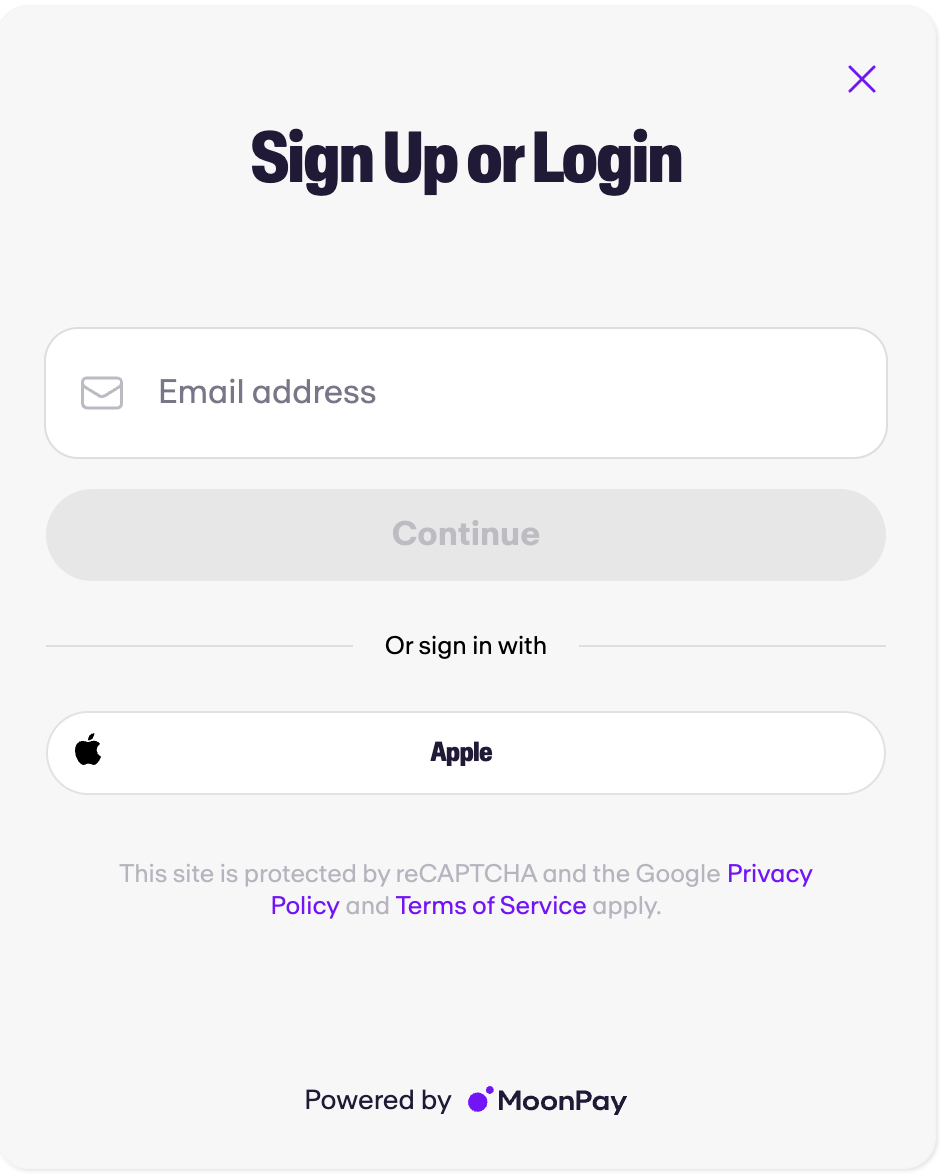
Linking wallet
The MoonPay Authenticate SDK offers the capability for users to seamlessly link their MetaMask wallet during the login process. This integration enhances user experience by providing direct access to wallet functionalities post-authentication.
Configuration
To enable the wallet linking feature in your SDK, incorporate the linkVerifiedWallet
option:
const sdk = new MoonPayAuthSDK('your_publishable_key', {
linkVerifiedWallet: true, // Enables the option for users to link their MetaMask wallet.
})
Once this option is set, users will have the option to link their MetaMask wallet when they log in through the SDK.
Try out the demo here: Demo
Screenshots
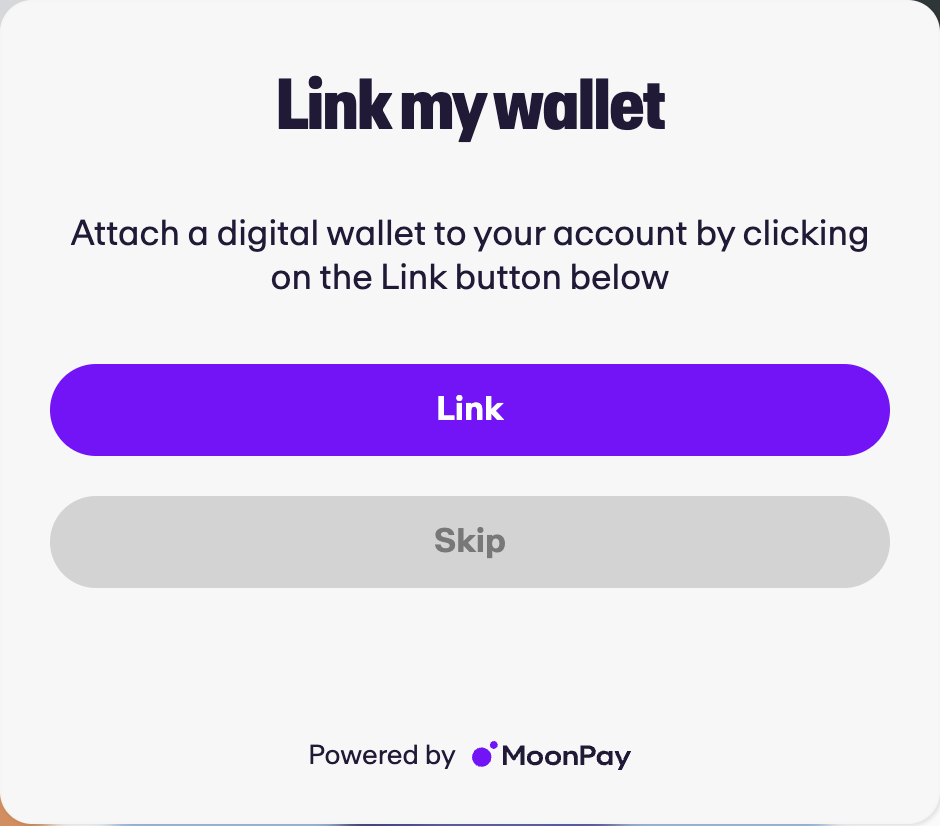
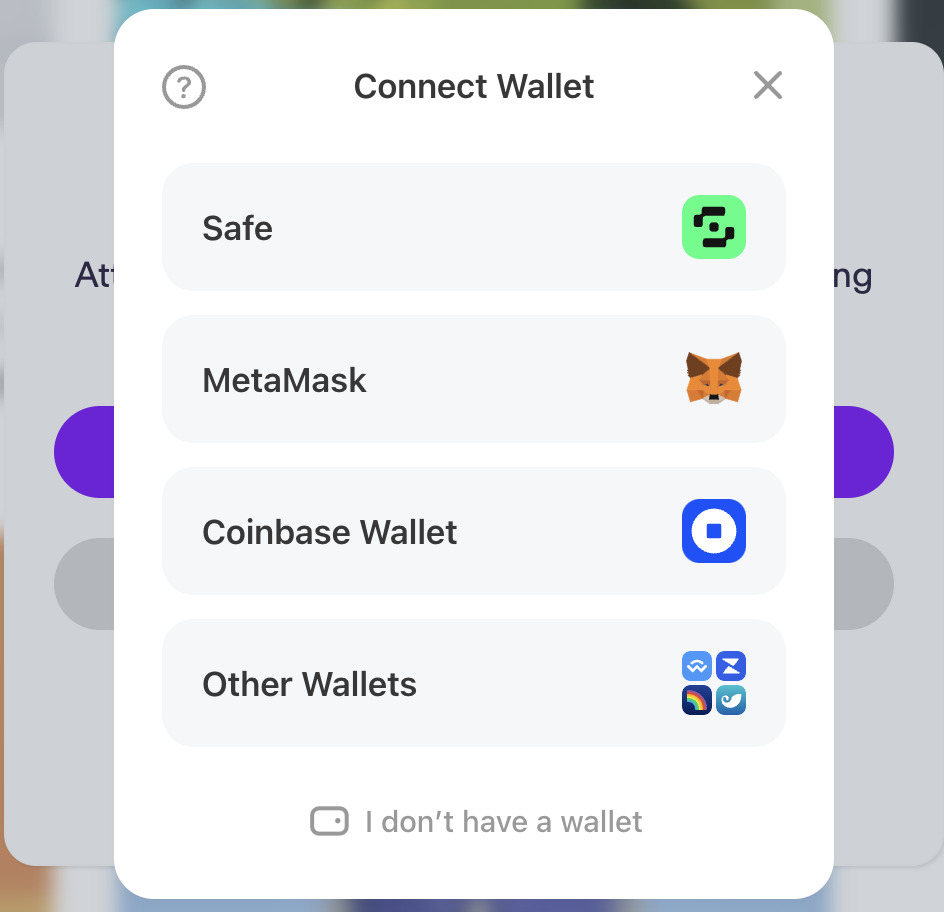
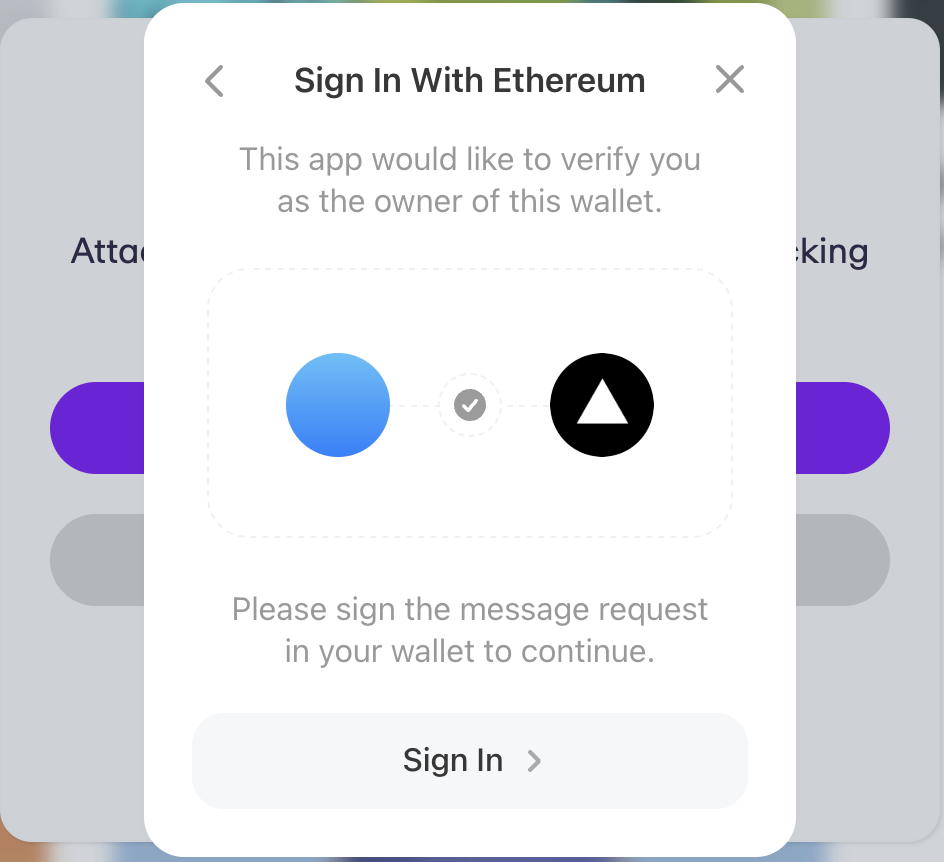
Updated 4 months ago