Distributing Passes
After creating an Apple or Google Wallet pass with POST /v0/passes
,
you will want to prompt the user to add the pass to their device. Our API provides you with an easy to use solution for distributing your created passes to be installed.
Info
Apple Wallet passes are binary files, while Google passes are cloud-hosted. Due to this distinction, we provide an additional
buffer
property for the file. This can be utilized to distribute the pass via email or SMS attachments.
Sample distribution payload:
"distribution": {
"google": {
"url": "https://api.ethpass.xyz/api/link/D9VgVhxABvRJdEuCuCKGNY1le964CZzB"
},
"apple": {
"url": "https://api.ethpass.xyz/api/link/99NoxIDVCSoARhraVTEcfXEH0FqrTbX1",
"buffer": {
"type": "Buffer",
"data": [80, 75, 3, 4, 10, 0, 0, 0, 0, 0, ...]
},
},
}
Working With the File Buffer
Your language of choice should have a library or built-in method for working with file buffers. In Javascript, you can use the built-in method like so:
For more on this, you can reference our email example below.
const buffer = {
"type": "Buffer",
"data": [80, 75, 3, 4, 10, 0, 0, 0, 0, 0, ...]
};
const file = Buffer.from(buffer.data).toString("base64");
Obtaining Distribution Data
You can obtain distribution data in two ways:
1. Response from POST /v0/passes
:
POST /v0/passes
:const body = {...};
// Create pass
const response = await fetch("https://api.ethpass.xyz/api/v0/passes", {
method: "POST",
body: JSON.stringify(body),
headers: new Headers({
"content-type": "application/json",
"X-API-KEY": "YOUR_SECRET_API_KEY"
}),
});
// Extract distribution info from result
const { id, distribution } = await response.json();
2. Querying with GET /v0/passes/{passId}/distribute
GET /v0/passes/{passId}/distribute
const passId = "5450c91b-9929-46a5-a2cc-3bbe7e728a45";
const response = await fetch(
`https://api.ethpass.xyz/api/v0/passes/${passId}/distribute`,
{
method: "POST",
body: JSON.stringify(body),
headers: new Headers({
"content-type": "application/json",
"X-API-KEY": "YOUR_SECRET_API_KEY",
}),
}
);
const { distribution } = await response.json();
Distribution Examples
Once you have the distribution data, you can expose it to a user in a number of ways:
- Download upon scanning a QR code
- Direct download
- Text
Download Pass via QR Code
An easy and mobile-friendly way to prompt users to download their pass is with a QR code.
You can use an open source tool like qrcode
to generate a barcode from the distribution URL.
If the user scans the QR code with their device, they will be routed to the distribution URL and prompted to install the pass into their wallet.
Please note: this example relies solely on the URL, and should only be used when a pass is first created. After the initial creation period, you may need to work with the file buffer.
// EXAMPLE IN REACT
import QRCode from "qrcode";
import { useEffect, useState } from "react";
const DownloadQRCode = () => {
const fileURL =
"https://api.ethpass.xyz/api/link/D9VgVhxABvRJdEuCuCKGNY1le964CZuG;
const [qrCode, setQrCode] = useState();
// Generate QR code from URL
useEffect(() => {
QRCode.toDataURL(fileURL, (err, url) => {
if (!err) setQrCode(url);
});
}, []);
// Render QR code
return <img src={qrCode} alt="QR Code" width={250} height={250} />;
};
Direct Download
To prompt a direct download of a pass, you can expose a button which will route to the distribution URL.
Please note: this example relies solely on the URL, and should only be used when a pass is first created. After the initial creation period, you may need to work with the file buffer.
// EXAMPLE IN REACT
const DirectDownload = () => {
const fileURL =
"https://api.ethpass.xyz/api/download/T3EPFmwiA9K2dy17swY6FbHnWBn7N83D";
return (
<a target="_blank" href={fileURL} rel="noreferrer">
Click Here to Add to Your Wallet
</a>
);
};
Email
If you would like to send email confirmations to pass holders, you can prompt users to download passes via email.
As an important note, Google Wallet and Apple Wallet passes are implemented differently, so the below example takes this into account.
Our example uses sendgrid to send emails (requires an API key).
// EXAMPLE IN JAVASCRIPT
import sgMail from "@sendgrid/mail"
const sendEmail = () => {
// Obtained from POST v0/passes or GET /v0/passes/{passId}/distribute
const fileURL = "https://api.ethpass.xyz/api/download/T3EPFmwiA9K2dy17swY6FbHnWBn7N83D";
const buffer = {
type: "Buffer",
data: [80, 75, 3, 4, 10, 0, 0, 0, 0, 0, ...]
};
// Your Information
const recipient = "[email protected]";
const sender = "[email protected]"; // Use a verified sender
const eventName = "Your Event";
// Email Details
let html = ""; // Google passes are URLs, can be included in HTML
let attachments = []; // Apple passes are files, and must be attached
if (platform === "apple") {
attachments = [
{
content: Buffer.from(buffer.data).toString("base64"),
filename: `${eventName}.pkpass`,
type: "application/vnd.apple.pkpass",
disposition: "attachment",
},
];
} else if (platform === "google") {
html = `<a href="${fileURL}">Add Pass To Your Wallet</a>`;
}
const msg = {
to: recipient,
from: sender,
subject: `${eventName}: Download Your Tickets`,
html,
attachments
}
return sgMail.send(msg);
}
Assets
We are happy to supply these assets for your Add to Wallet buttons:
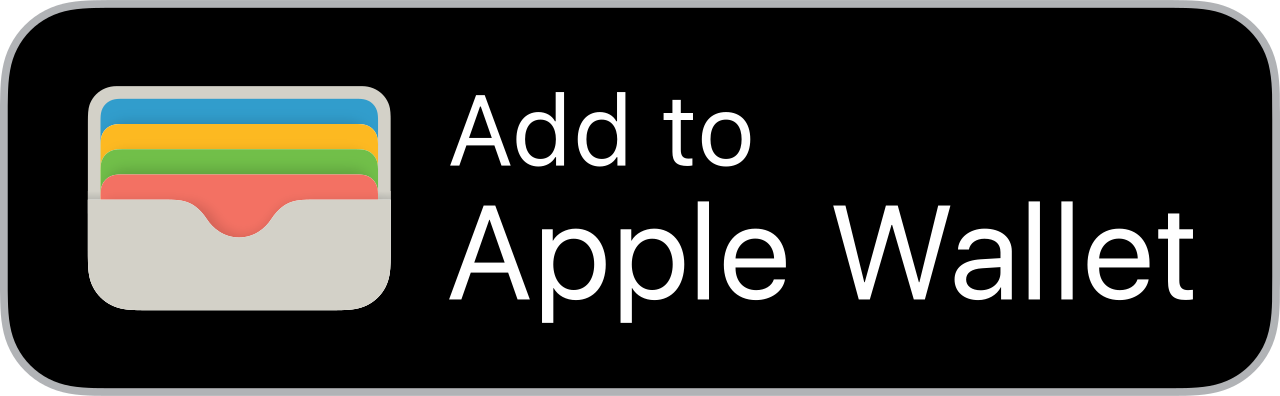

Updated 2 months ago